本帖最后由 wwwwwllllk 于 2023-4-10 13:25 编辑
本帖最后由 wwwwwllllk 于 2023-4-9 16:01 编辑
本帖最后由 wwwwwllllk 于 2023-4-9 11:41 编辑
直接通过代码举例子吧
const [count, setCount] = useState(1)
function add() {
setCount(count+1)
console.log(count) //输出应该是1
}
// 因为setState函数式异步的,但是恰好我们要在别的函数用的count肯定是最新的这个时候我们怎么做
useEffect(() => {
// 在这里执行之后的操作
}, [count])
// 但是其实我们使用useEffect在真实的项目中这个监听的变量是应该经过仔细思考以后再做决定的,当这个变量在多个地方都会触发变化时, 它都会触发useEffect里面的函数,所以你的业务是否是这样的
我们可以写setState回调拿到你想要的那个值。
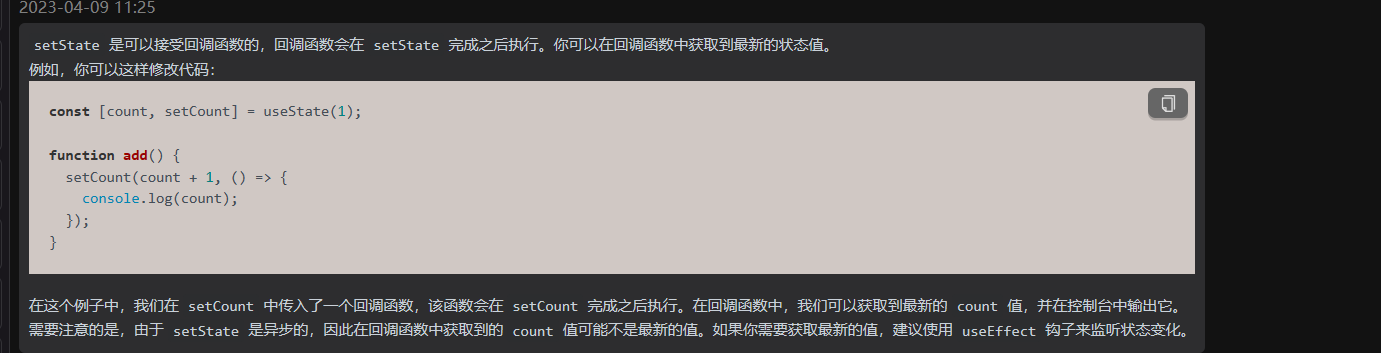
或者我们就是直接通过传参把函数传过去
const [count, setCount] = useState(1)
function add() {
setCount(count+1)
console.log(count) //输出应该是1
reduce()
}
function reduce() {
setCount(count-1) // 我肯定是希望它是2-1,因为add先加了1
console.log(count)
}
第一种写法(压根就不要用useState)
function add() {
let count = 1
count = 1+1
reduce(count)
}
function reduce(count) {
let count = count-1 // 我肯定是希望它是2-1,因为add先加了1
console.log(count)
}
第二种
const [count, setCount] = useState(1)
function add() {
setCount(count+1)
console.log(count) //输出应该是1
}
useEffect(() => {
reduce() // 这个时候coutn变化就会触发useEffect函数
}, [count])
function reduce() {
setCount(count-1) // 我肯定是希望它是2-1,因为add先加了1
}
第三种
const [count, setCount] = useState(1)
function add() {
setCount(count+1, () => {
reduce(count+1)
})
}
function reduce(count) {
setCount(count-1) // 我肯定是希望它是2-1,因为add先加了1
}
第四种(最喜欢的)
const [count, setCount] = useState(1)
function add() {
setCount(count+1)
setTimeout(() => {
reduce()
}, 0)
}
function reduce() {
setCount(count-1) // 我肯定是希望它是2-1,因为add先加了1
console.log(count)
}
第四种好像是错的

浏览器执行异步代码, 浏览器会先执行同步代码,然后把异步代码放到异步队列再执行,
我之前以为,异步队列执行是按照顺序的,这个执行完成拿到结果再执行下一个,但是好像不是的,异步队列按照顺序执行,但是不一定第一个结果返回的比第二结果快,所以第四种方法好像是错的
我之前理解错了
setCount(2)
setList([{name:'zhangsan'}])
useEffect(() => {
console.log(count) // 这里的count应该是2
}, [list])
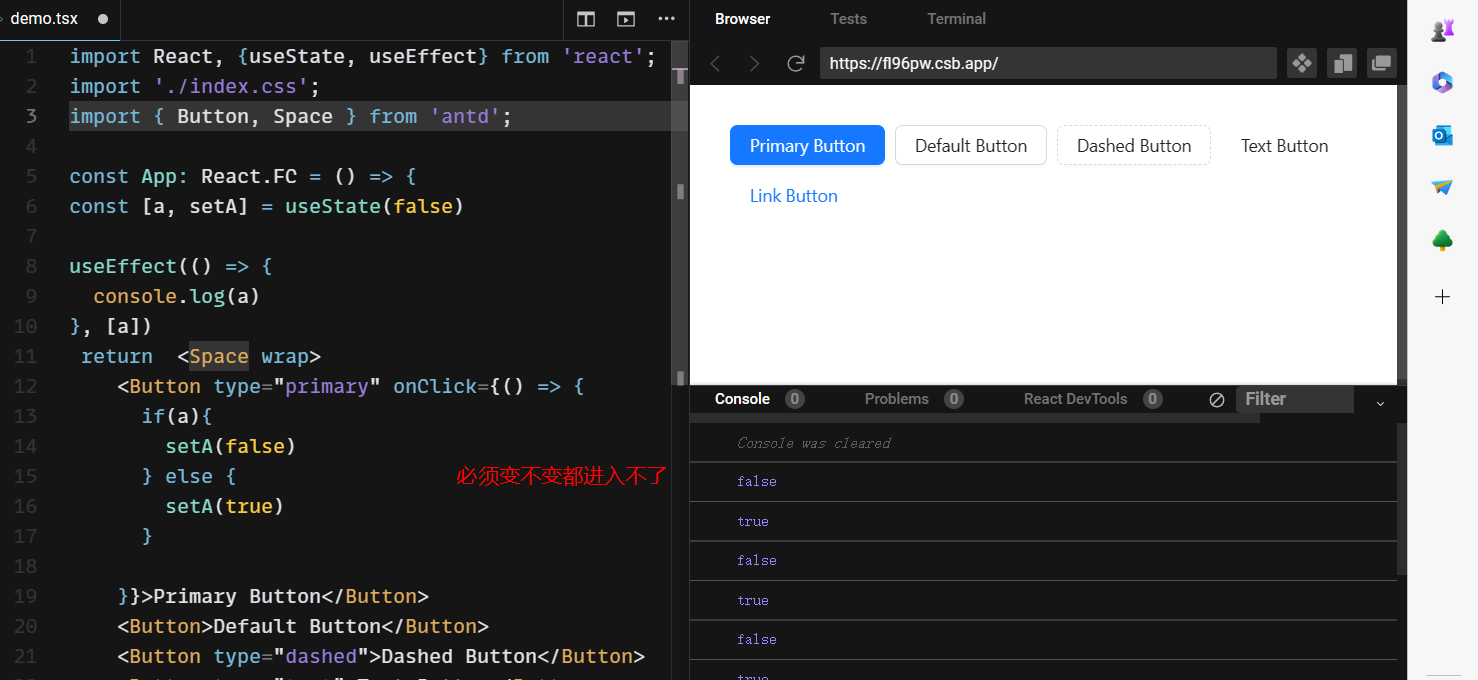
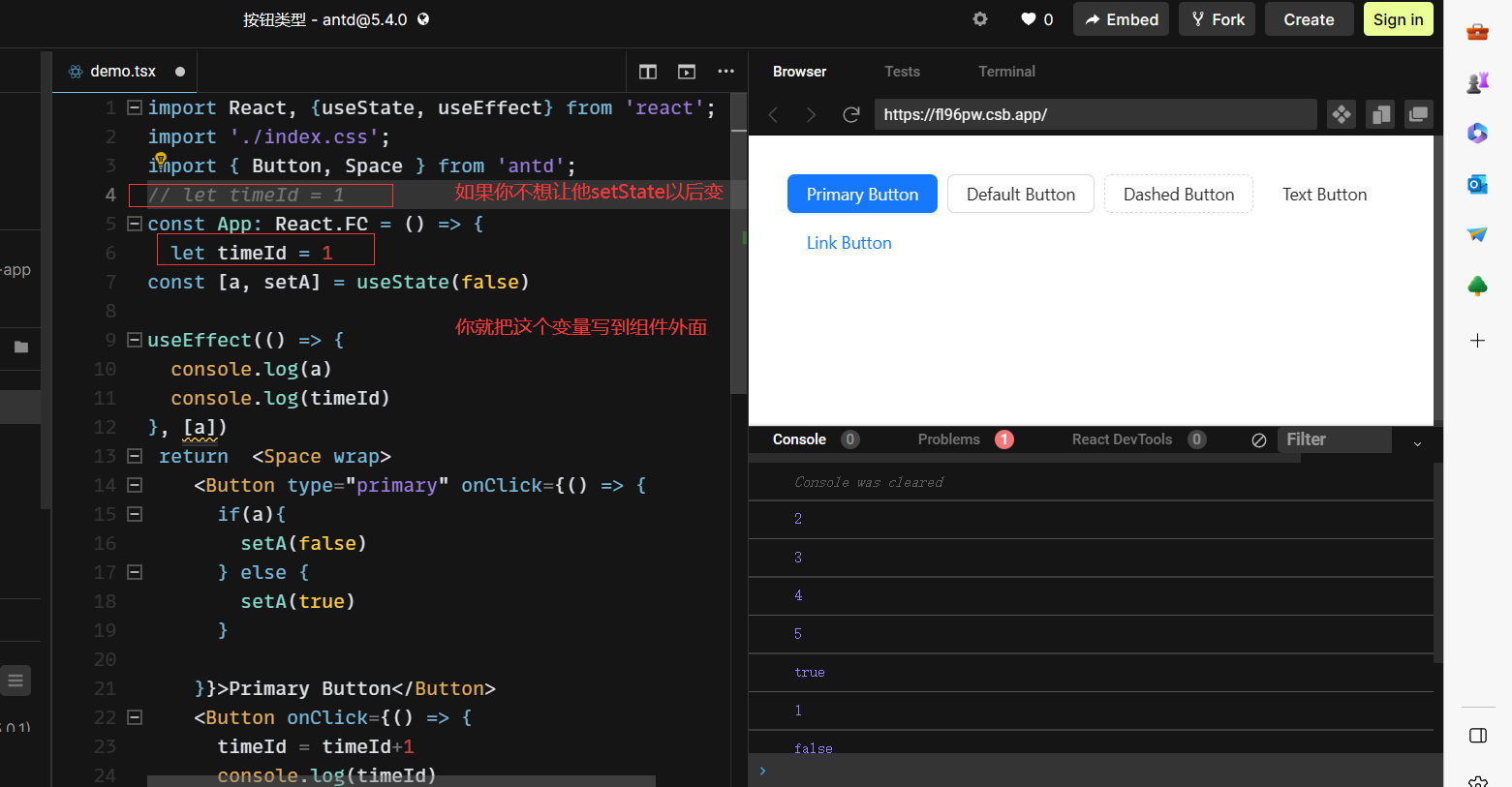
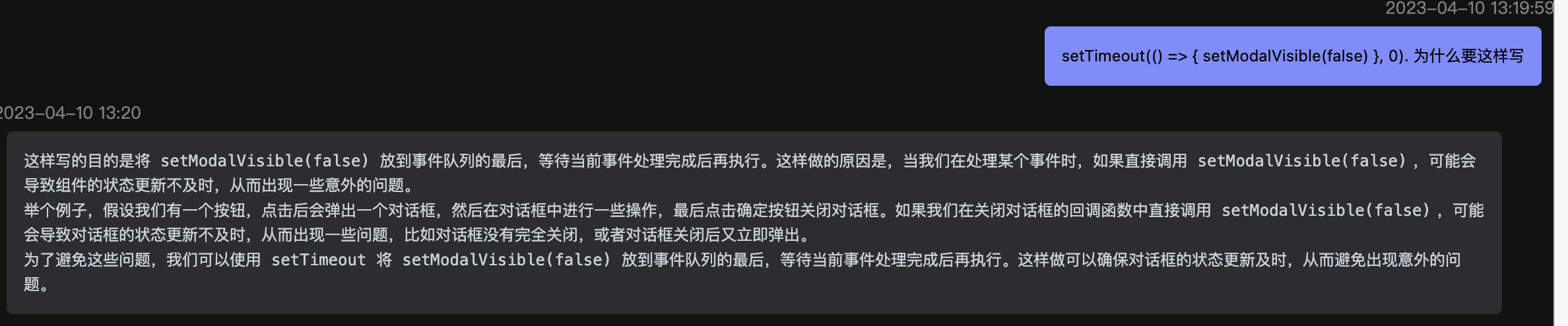